Roop was wondering how to access an (QScript) array from C++. I spent sometime trying to figure how to do that, so I thought I will let the world know.
A search on the QScriptValue documentation page yields QScriptValue::isArray(). We can use that to determine if the value is an array or not. Unfortunately, it doesn’t really state there how to access contents of the array. My initial reaction was to just convert the value to a QVariant (using toVariant()) and then convert the variant to a QVariantList (toList()). That didn’t quite work.
The answer lies in understanding arrays and objects in EcmaScript – Everything is an object. Every object is really a map from properties to values. What that means is that an EcmaScript array is just an object. And the values you put into it are just properties of the array. The [] syntax is EcmaScript is just to make us feel at home and make us believe you are working with arrays and not some fancy objects. So,
array[1] = “foo”; // creates property named “1″ with value “foo”
With that revelation, you should be able to find QScriptValue::property(). Just pass the values 0, 1, 2 and so on as arrayIndex and you should receive the values. But, what about the length of the array? Remember, everything is a property in an object. The length is stored in the length property of the array which you can query using the other QScriptValue::property() overload.
P.S 1:
Note that, array indices are not limited to integers (it is a map). So, the below is perfectly fine.
array[“one”] = “foo;
I can imagine it to be common to inspect all the properties of an object. You can use QScriptValueIterator for that.
P.S 2:
array[1] is the same thing as array[“1”]. The keys in EcmaScript maps are strings.
Update
Roop found a much much easier way to process arrays: qScriptValueToSequence
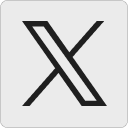