I don’t get exceptions. There’s this theory that they should be raised for ‘exceptional’ cases. But in reality lots of API uses it as a way to just pass back the error code. Joel has already said whatever I want to say about exceptions over a decade ago, so I won’t repeat his article here.
The best abuse of exceptions can be seen in APIs like JSON.parse, JSON.stringify and most node.js sync functions. It’s annoying because the cases are hardly exceptional. And to simply check if a parse succeeded, you have to surround your code with try/catch.
Safety Dance
To remedy the situation, I have a written a module called Safety Dance. The idea is simple – you can use the safeCall() function to wrap exception throwing functions. If safeCall() catches an exception, it will make the function return null or false (or whatever error value makes sense for that API call).
For example,
var safetydance = require('safetydance'), safeCall = safetydance.safeCall; var result = safeCall(function () { return 1 + 2; }); // will return 3 result = safeCall(function () { throw new Error('bad'); }); // will return null safetydance.error; // contains the error 'bad'
There are convenience wrappers for some API. For example,
try { var obj = JSON.parse(str); } catch (e) { }
becomes
var obj = safe.JSON.parse(str); if (obj === null) { // safe.error contains the actual error }
At this point, someone will point out that null is valid JSON and they would be correct. But I am yet to see any REST API that uses null as a valid API result. In any case, you can write safe.safeCall(function() { JSON.parse(str); }, undefined);. The second parameter is the value to return if safeCall() catches an exception.
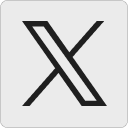